5 Tips for Improving Database Performance in Django
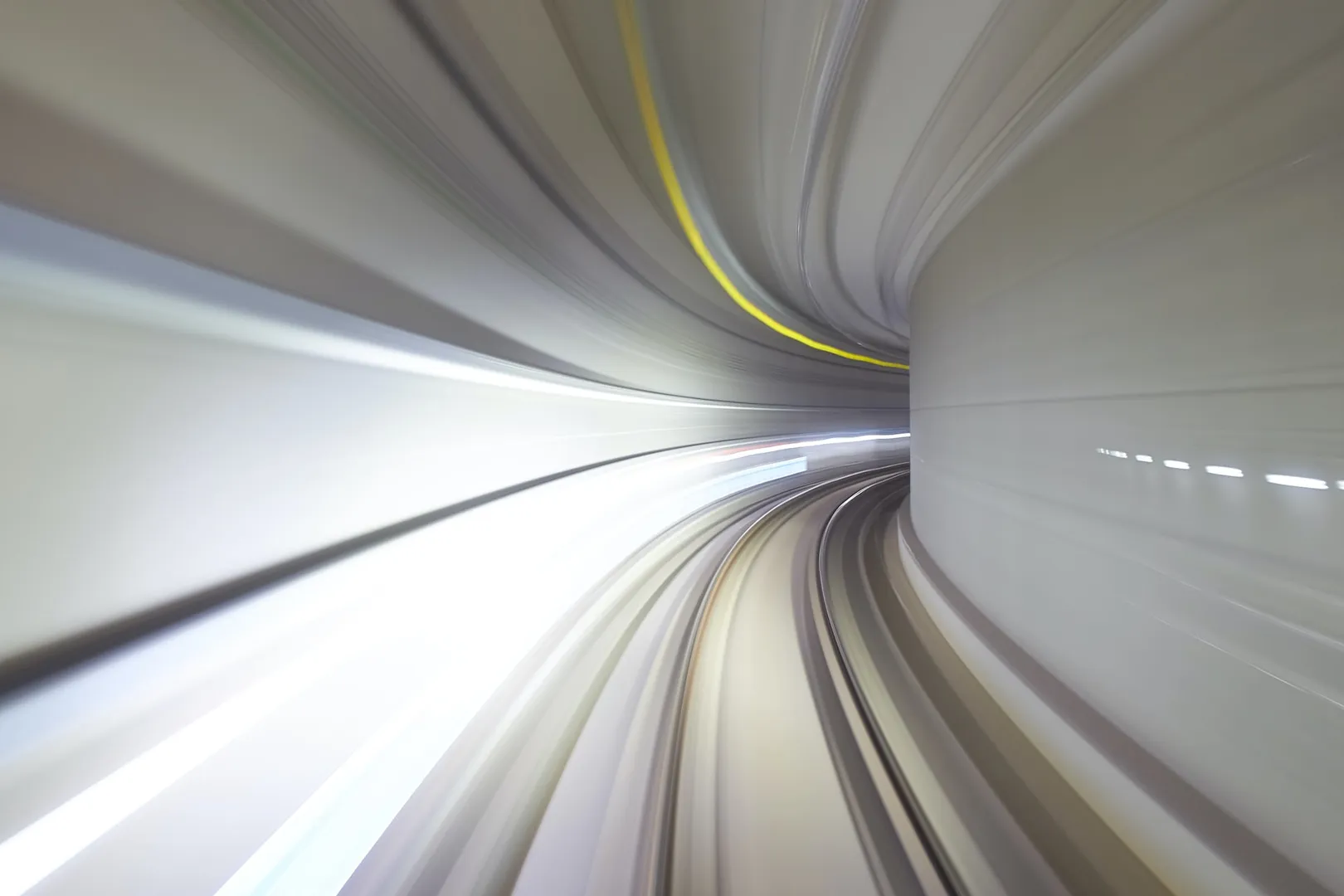
Optimizing the performance of a Django project with a SQL database is essential to ensure smooth operation and a great user experience. This post explores five techniques for optimizing the performance of your Django SQL database.
database django optimization performance sql
Optimizing the performance of a Django project with a SQL database can be a daunting task, but by improving the speed and efficiency of your database queries, you can ensure that your application runs smoothly and provides a great user experience. In this post, we will explore five techniques for optimizing the performance of your Django SQL database:
-
Use the right database engine. Django uses SQLite as its default database, which is a lightweight and easy-to-use SQL database engine. It also supports several different SQL databases, and each one has its own strengths and weaknesses. For example, MySQL is widely used and has a large community, but it may not be the best choice for large-scale applications. On the other hand, PostgreSQL offers more advanced features and can handle larger volumes of data, but it may be more difficult to set up and use.
-
Proper table design and organization: The structure of your database tables has a big impact on query performance. To optimize the performance of your queries, it's important to carefully choose data types and organize columns in the most effective way. This can help minimize data redundancy and ensure that your queries run as efficiently as possible.
-
Choose appropriate data types: Use the most appropriate data type for each column to minimize storage space and improve query performance. For example, use smaller integer types instead of larger ones if possible, and use
models.CharField(...)
instead ofmodels.TextField(...)
for columns with fixed-length data. -
Normalize your data: Normalization is the process of organizing data into a logical structure to minimize data redundancy and improve data consistency. Use normalization techniques to organize your tables into logical groupings and reduce the number of duplicate data.
-
Partition large tables: If you have tables with a large number of rows, consider partitioning them into smaller, more manageable pieces. This can help improve query performance by reducing the amount of data that needs to be scanned for each query.
-
Use denormalization carefully: While normalization can help improve query performance, denormalization can sometimes be used to improve performance for certain queries. However, it can also increase data redundancy and make it harder to maintain data consistency.
-
-
Regular monitoring and analysis: To ensure that your database queries are performing optimally, it's important to regularly monitor and analyze their performance. This can help you identify any areas where queries are running slowly, and take steps to improve their performance. Tools like database profilers can be useful for this purpose, as they allow you to identify slow-running queries and determine the underlying causes of their performance issues.
-
Use of caching: Caching can be a useful technique for improving the performance of your SQL database. By storing frequently accessed data in a cache, you can reduce the number of times your application needs to query the database, which can help speed up your application and improve its overall performance. Django provides a caching framework that allows you to cache the results of queries using a variety of cache backends, including in-memory caches, file-based caches, and remote caches like Redis. We'll go deeper into Redis in a future post.
-
Use of indexes: Indexes can help speed up query performance by allowing the database to quickly locate the specific data it needs. However, it's important to use indexes wisely, as overusing them can actually have a negative impact on performance. To determine which columns to index, you can use tools like
EXPLAIN
to analyze the performance of your queries and identify which columns are frequently used in filters andWHERE
clauses. You can easily access to this tool installing Django Debug Toolbar app in your project.
This is an example of how to create an index for a Django model:
from django.db import models
class MyModel(models.Model):
# define your model fields here
my_field = models.CharField(max_length=64)
# create an index on the "my_field" field
class Meta:
indexes = [
models.Index(fields=['my_field'])
]
Remember to create a new migration after adding a new index.
In conclusion, optimizing the performance of your Django database is an important task that can help ensure the smooth operation of your application. By following these five tips, you can ensure that your database is running efficiently and effectively, providing a better experience for your users and increasing productivity for your team.