Time series class prototype generation for classification with DBA
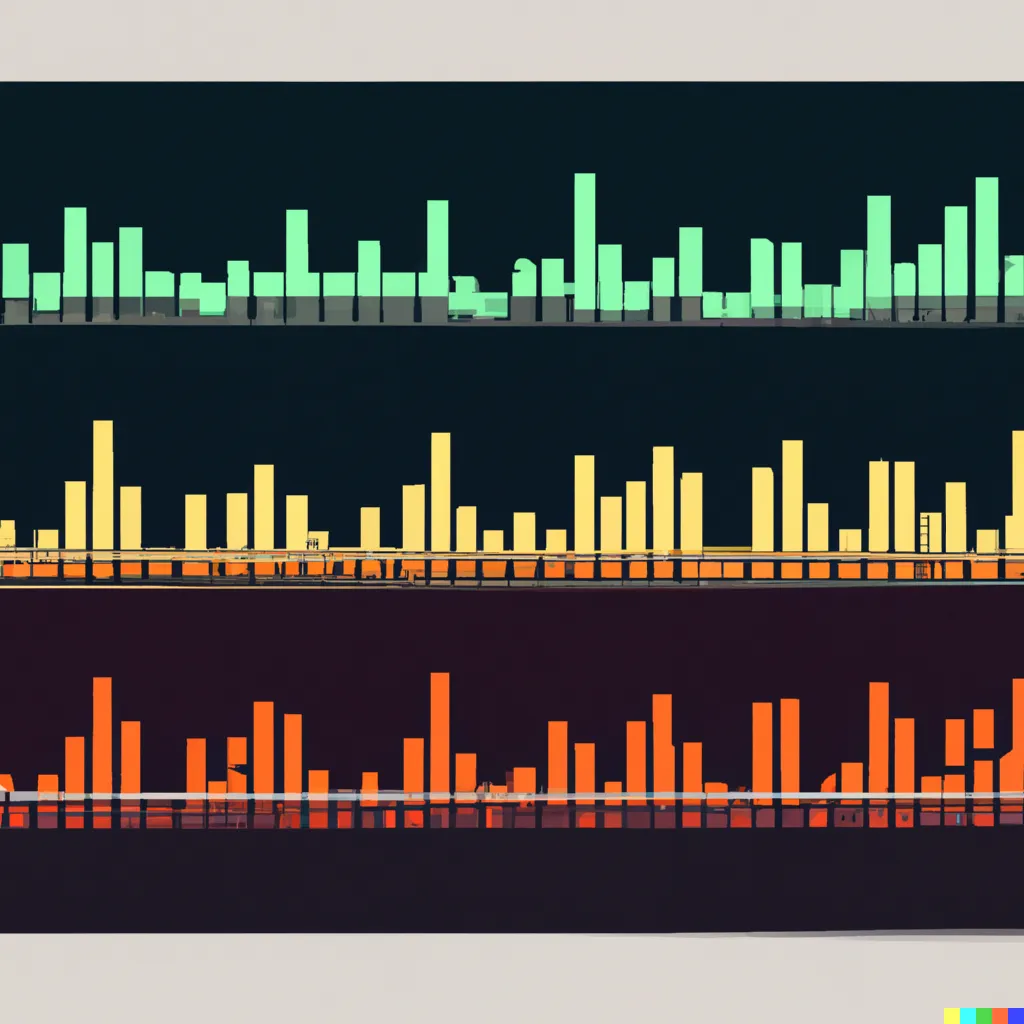
Time series classification is an important task in many fields, such as finance, healthcare, and meteorology.
A common approach to time series classification is to use class prototypes generated by a time series “averaging algorithm”. Each of the classes is represented by a class prototype, which is typically the "average" time series for that cluster. These class prototypes can then be used to classify new time series data.
In this post, we will explore the use of class prototypes for time series classification and discuss some of the advantages and challenges of this approach.
machine learning time-series
Time series classification is an important tool in a variety of fields, as it allows researchers and practitioners to analyze and understand data that changes over time.
This type of analysis is particularly useful in fields such as finance, where understanding trends and patterns in stock prices can help investors make informed decisions, or healthcare, where time series classification can be used to analyze medical data and detect potential issues or trends in patient health.
Overall, the ability to classify and analyze time series data is crucial for making informed decisions and predictions in a wide range of fields such as meteorology and climate science among others.
Classification Task
One advantage of using class prototypes generated by an averaging algorithm for time series classification is that the prototypes can capture the inherent structure and variability of the time series data, which can improve the accuracy of the classification results.
The classification task is the following:
- Generate class prototypes for each class with an averaging algorithm.
- For each time series in the classification dataset, calculate the distance between the time series and each class prototype using a distance measure such as the DTW distance.
- Assign the time series to the cluster with the closest class prototype.
- Repeat this process for all time series in the classification dataset.
This process can be repeated for different averaging algorithms and different distance measures to find the combination that produces the most accurate classification results. Once the best combination has been identified, the class prototypes can be used to classify new time series data.
DTW Barycenter Averaging (DBA)
The dynamic time warping (DTW) barycenter algorithm is a method for averaging time series data. It is based on the dynamic time warping (DTW) distance measure, which calculates the similarity between two time series by aligning them in time.
The DTW barycenter algorithm uses this distance measure to cluster time series data by iteratively updating a central point, or barycenter, for each cluster. The barycenter is updated by computing the average DTW distance between all time series in the cluster and the current barycenter, and then selecting the time series that minimizes this average distance as the new barycenter.
This process is repeated until the barycenters converge, at which point the algorithm terminates and the resulting clusters can be used for further analysis or visualization.
One advantage of the DTW barycenter algorithm is that it is able to handle time series data with different lengths and variations in tempo, which can be challenging for other averaging methods. Additionally, because the DTW distance measure is based on the alignment of time series, it is able to capture more complex relationships between time series than methods that rely on simple distance measures.
Classification example
Suppose we have a dataset of heart rate time series data collected from different individuals, and we want to classify the individuals into two groups: healthy and unhealthy.
First, we can use the DBA algorithm to cluster the time series data into two groups. This will generate class prototypes for each group, which will represent the "average" heart rate time series for that group.
Next, we can calculate the DTW distance between each time series in the classification dataset and the class prototypes for each group. The time series will be assigned to the group with the closest class prototype.
For example, suppose the DBA algorithm generates the following class prototypes for the two groups:
- Group 1: a slowly varying heart rate time series with an average rate of 70 beats per minute
- Group 2: a rapidly varying heart rate time series with an average rate of 100 beats per minute
If we calculate the DTW distance between a particular time series and these class prototypes, and find that it is closer to the prototype for Group 1, we would classify that time series as belonging to the healthy group. If it is closer to the prototype for Group 2, we would classify it as belonging to the unhealthy group.
This is a simple example of time series classification using class prototypes generated with the DBA algorithm. In a more complex classification task, we would likely use more sophisticated algorithms and more features to improve the accuracy of the classification results.
Python toy example
# Import necessary libraries and modules
import numpy as np
from tslearn.barycenters import dtw_barycenter_averaging
from dtaidistance import dtw
# Define the time series data for each class
# Set the seed for reproducibility
np.random.seed(0)
# Generate 5 time series for each class
class1_ts = [np.random.random(10) for _ in range(5)]
class2_ts = [np.random.random(10) for _ in range(5)]
class3_ts = [np.random.random(10) for _ in range(5)]
# Compute the barycenter for each class using dtw_barycenter_averaging
class1_barycenter = dtw_barycenter_averaging(class1_ts)
class2_barycenter = dtw_barycenter_averaging(class2_ts)
class3_barycenter = dtw_barycenter_averaging(class3_ts)
# Define a new time series and compute its distance to each barycenter
new_time_series = [1, 2, 3, 4, 5, 6, 7]
distance_class1 = dtw.distance(new_time_series, barycenter_class1)
distance_class2 = dtw.distance(new_time_series, barycenter_class2)
distance_class3 = dtw.distance(new_time_series, barycenter_class3)
# Classify the new time series based on its similarity to the barycenters
# Create a list of barycenters
barycenters = [class1_barycenter, class2_barycenter, class3_barycenter]
# Initialize minimum DTW distance and corresponding class
min_dtw = float("inf")
min_class = None
# Iterate over barycenters and their indices
for i, barycenter in enumerate(barycenters):
# Calculate DTW distance
dtw_distance = dtw(new_ts, barycenter)
# Update minimum DTW distance and corresponding class if necessary
if dtw_distance < min_dtw:
min_dtw = dtw_distance
min_class = i
# Print the most similar class
print("New time series is most similar to class", min_class + 1)
This code uses the tslearn.barycenters.dtw_barycenter_averaging method to generate an average time series for each of three different classes. It then defines a new time series and computes the distance between this time series and each of the generated barycenters using DTW as the distance measure.
Finally, the code classifies the new time series based on its similarity to the barycenters, and prints the class that it belongs to. This simple example demonstrates how to use DTW and the dtw_barycenter_averaging method to average and classify time series data.
A final word
In conclusion, class prototypes can be a powerful tool for time series classification. They capture the inherent structure and variability of the time series data.
This is just one example of how to classify time series data using DBA and DTW, and there are many other algorithms and techniques that can be used for this purpose.
In future posts, we will explore other methods for time series classification and discuss their relative strengths and weaknesses. Stay tuned!